React Full Stack Development Course
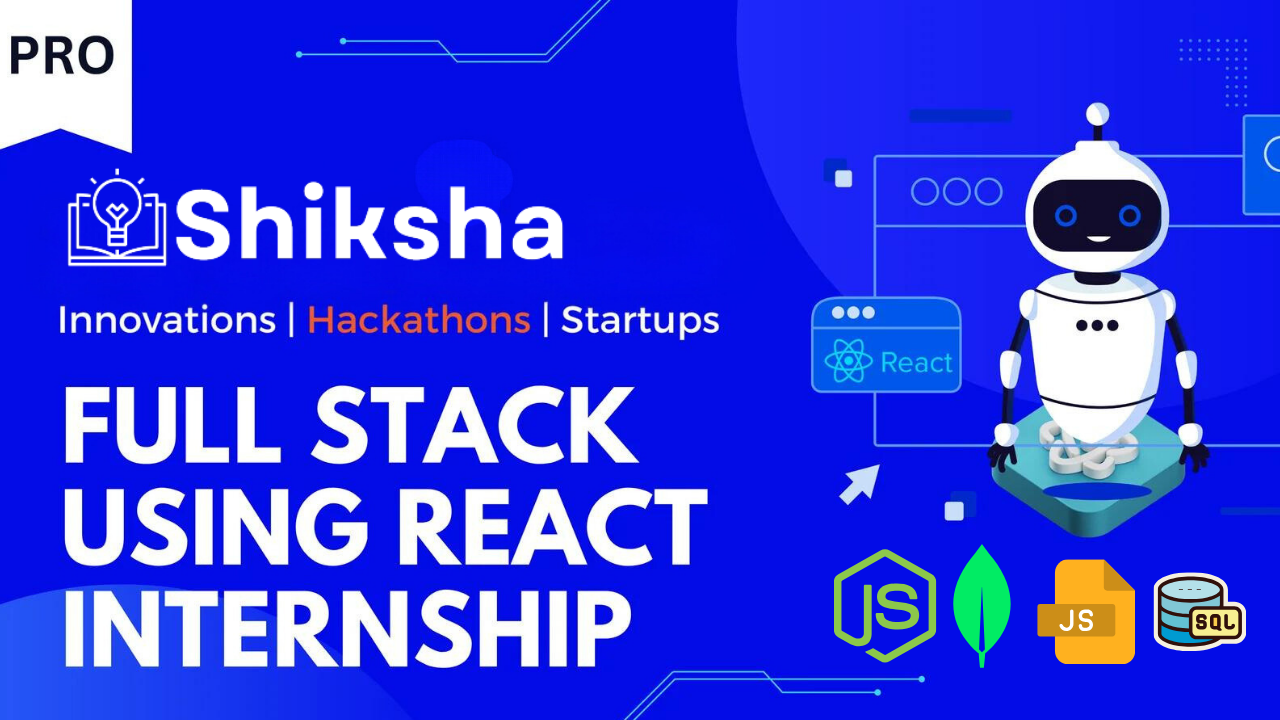
About Course
Course Overview This course is designed for developers who want to master full-stack development using React, one of the most popular JavaScript libraries for building user interfaces. The course covers the entire development process, from setting up the environment to deploying the application. You’ll gain hands-on experience by building real-world projects with front-end and back-end components. Target Audience This course is ideal for:
- Frontend developers were looking to expand their skills to backend development.
- Backend developers wanting to learn modern frontend development with React.
- Full stack developers aiming to solidify their knowledge in both areas.
- Students and professionals seeking to build dynamic and interactive web applications.
- Prerequisites Before starting this course, you should have a basic understanding of HTML, CSS, and JavaScript. Familiarity with Node.js will be helpful but is not required. If you’re
- completely new to JavaScript or web development, consider starting with an introductory course before diving into full stack development.
Course Content Module 1: Introduction to React In this module, you will learn the basics of React, including how to set up a React project, understand components, state, and props, and create your first React application.
- Setting up the development environment
- Understanding JSX and components
- State and props in React
- Handling events and forms
- Conditional rendering
Module 2: Advanced React Concepts This module delves deeper into React by introducing hooks, context API, and routing. You’ll learn how to manage the application state and navigate between different components.
- React Hooks (useState, useEffect, useContext)
- Context API for state management
- React Router for client-side routing
- Advanced component patterns
Module 3: Backend Development with Node.js and Express Moving to the backend, you will learn how to set up a Node.js server and build RESTful APIs using Express. This module will cover essential backend concepts, including routing, middleware, and database interactions.
- Introduction to Node.js and Express
- Building RESTful APIs
- Working with databases (MongoDB)
- Authentication and authorization
- Error handling and logging
Module 4: Connecting Frontend and Backend In this module, you’ll learn how to integrate the React frontend with the Express backend. You’ll create a full-stack application where the front end communicates with the backend via RESTful APIs.
- Fetching data from the backend using Axios or Fetch API
- Managing application state with React and Redux
- Handling authentication in a full-stack app
- Deploying a full stack application
Module 5: Project Development The final module is a hands-on project where you’ll apply everything you’ve learned to build a fully functional full-stack application. This could be a blog platform, e-commerce site, or other web app that interests you.
- Planning the project architecture
- Setting up the project repository
- Implementing core features (CRUD operations, user authentication, etc.)
- Testing and debugging
- Final deployment and hosting
Tools and Technologies Throughout the course, you will use various tools and technologies that are crucial for full-stack development:
- React: Frontend JavaScript library for building user interfaces.
- Node.js: JavaScript runtime for building server-side applications.
- Express: Web framework for Node.js to build RESTful APIs.
- MongoDB: NoSQL database for storing application data.
- Git & GitHub: Version control and code collaboration tools.
- Webpack & Babel: Module bundler and JavaScript compiler/transpiler.
- Heroku/Vercel: Platforms for deploying full-stack applications.
Course Duration This course is designed to be completed in 10 weeks, with an estimated commitment of 5-10 hours per week. The course is self-paced, allowing you to progress at your own speed. Each module includes a mix of video lectures, reading materials, coding exercises, and quizzes to reinforce your learning. Course Benefits By the end of this course, you will:
- Have a solid understanding of full-stack development using React and Node.js.
- Be able to build and deploy dynamic web applications from scratch.
- Gain hands-on experience with real-world projects.
- Improve your problem-solving and coding skills.
- Earn a certificate of completion to showcase your new skills.
Course Content
Introduction
-
Intro
01:29 -
Installing Node JS
01:44 -
IDE
01:38